Object-Oriented Programming in Javascript
The first and very important question, is JavaScript really an object-oriented programming (OOP) language? In my personal opinion, I will say: NO at all; JavaScript, emulates object classes via constructor functions.
But first, what is Object-Oriented Programming?
A programming paradigm centered around objects rathen than functions.
Mosh is one of the best tutors I have ever met, and I don’t want to copy a ton of text, and I personally don’t want to explain and transcribe a lot of content that can be better understood through a video.
Also, this is a very interesting resource in Medium: A Guide to Object-Oriented Programming in JavaScript and of course this from Eric Ellioot Master the JavaScript Interview: What’s the Difference Between Class & Prototypal Inheritance?.
I made an easy example:
class Person {
constructor(firstName, age) {
this.firstName = firstName;
this.age = age;
}
static getAge(person) {
return person.age;
}
greeting() {
return `Hello ${this.firstName}`;
}
}
class Teacher extends Person {
constructor(firstName, age, id, seniority) {
super(firstName, age);
this.id = id;
this.seniority = seniority;
}
set teacherId(newId) {
this.id = newId;
}
get teacherId() {
return this.id;
}
}
And now, the most important part, how can I check if this works?
const newPerson = new Person('Andres', 35);
Person.getAge(newPerson); // 35
newPerson.greeting(); // "Hello Andres"
const newTeacher = new Teacher('SrHart', 53, 13579, 'Senior');
newTeacher.teacherId = 24680;
newTeacher.teacherId; // 24680
newTeacher.greeting(); // "Hello SrHart"
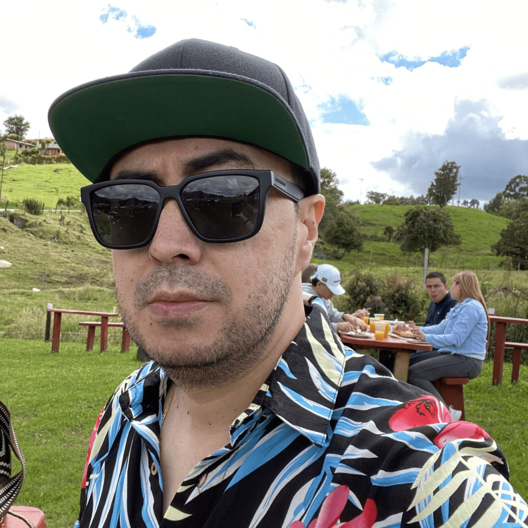
About Andrés Bedoya
JavaScript software engineer, internet enthusiast and blogger from an early age. He strongly believes in the free culture.
Learn more about Andrés Bedoya